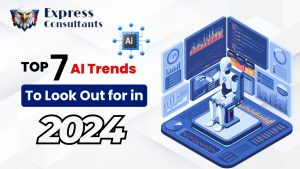
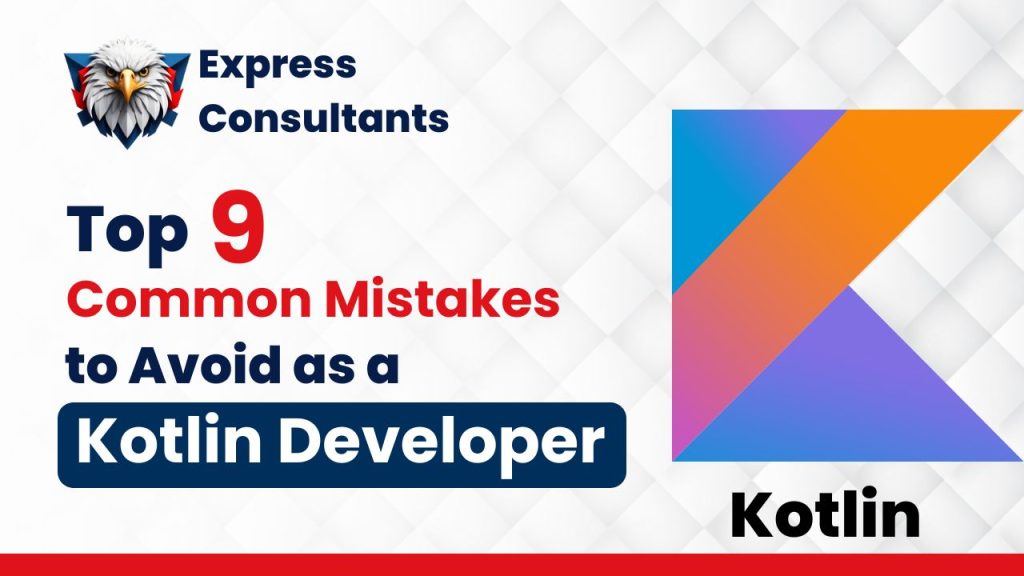
Top 9 Common Mistakes to Avoid as a Kotlin Developer
Top 9 Common Mistakes to Avoid as a Kotlin Developer
“By failing to prepare, you are preparing to fail.” – Benjamin Franklin. When it comes to coding in Kotlin, a lack of awareness about the language’s features can be just as damaging as skipping preparation altogether. Kotlin is one of the most popular languages for Android development, but that doesn’t mean every developer uses it to its full potential. Whether you’re transitioning from Java or just diving into Kotlin, it’s easy to make mistakes that hinder your progress. Let’s dive into some common mistakes to avoid as a Kotlin developer, so you can write better, cleaner code.
Mistake 1: Ignoring Null Safety Features
Kotlin’s null safety is one of its most touted features. It basically takes away the headache that most of us have from dealing with NullPointerException in Java. But believe it or not, some devs still manage to overlook this powerful feature. Sounds wild, right?
- In Kotlin, you have to specifically mark a variable as nullable by adding a ? to its type. If you’re not using this feature, you’re asking for trouble. Why? Because you’re treating Kotlin like Java, ignoring one of the very things that makes it better.
- Skipping null safety can result in bugs that are hard to debug and can cause unexpected crashes. For example, instead of handling nullables with care, developers might assume everything will work fine, only to get bitten by the dreaded runtime errors.
So, next time you write code, make sure you fully embrace Kotlin’s null safety tools like safe calls (?.), the Elvis operator (?:), and non-null assertions (!!).
Mistake 2: Overusing the !! Operator
The !! operator can be a double-edged sword. It’s tempting, I know. You see that pesky null, and you just slap a !! in there to make it disappear. Boom! Problem solved, right? Wrong. Overusing this operator is like playing with fire.
- The !! operator tells Kotlin, “I know this value isn’t null, let me handle it!” However, if the value is indeed null, guess what? Your app will crash with a KotlinNullPointerException. Essentially, it’s like running with scissors. Sure, you can do it, but you’re risking unnecessary issues.
Instead of recklessly throwing in !!, use safe calls and proper null checks. Kotlin gives you tools to manage nullability properly, so use them wisely.
Mistake 3: Not Using Extension Functions
Ah, extension functions. These bad boys are one of Kotlin’s coolest features, yet many developers forget to take full advantage of them. Extension functions let you extend the functionality of classes without modifying their source code. That’s pretty neat, right?
- For example, if you frequently need to transform a string to title case, instead of rewriting the same code everywhere, you can create an extension function:
fun String.toTitleCase(): String {
return this.split(” “).joinToString(” “) { it.capitalize() }
}
See? Now you can call .toTitleCase() on any string, making your code cleaner and more readable. Extension functions are an easy way to DRY (don’t repeat yourself) up your code. Don’t skip ‘em!
Mistake 4: Neglecting Data Classes
Data classes are your go-to when you just need to hold data. Kotlin makes this super easy with the data keyword, but somehow, devs still forget to use it!
- If you’re writing a class that’s just meant to hold data and you’re manually writing equals(), hashCode(), or toString() methods, you’re doing it wrong. Data classes automatically provide these methods, saving you tons of time and effort.
Why reinvent the wheel? Just declare a class as a data class, and Kotlin does the heavy lifting for you.
Mistake 5: Misusing Coroutines
Coroutines are what makes Kotlin a real treat for asynchronous programming, but they can easily be misused if you’re not careful. One common mistake? Launching coroutines on the wrong dispatcher or not managing them properly.
- Developers might launch coroutines on the main thread, resulting in UI blocking or slow performance. To avoid this, make sure you launch coroutines on the correct dispatcher, such as Dispatchers.IO for network or disk operations.
Coroutines are a fantastic tool, but if you misuse them, you might end up with unresponsive UIs or unexpected crashes. Always be mindful of where and how you’re launching your coroutines.
Mistake 6: Writing Java-Style Kotlin Code
Kotlin and Java are close relatives, but writing Kotlin like it’s Java? Big mistake. Many developers transitioning from Java tend to write Java-esque code in Kotlin. This defeats the purpose of using a modern, concise language like Kotlin.
- For example, using traditional loops (for loops) instead of taking advantage of Kotlin’s collection functions like map, filter, or forEach. Why write verbose code when Kotlin allows you to express it more succinctly?
Kotlin is designed to be expressive and concise, so don’t fall into the trap of writing Java-style code.
Mistake 7: Not Leveraging Kotlin’s Standard Library
If you’re not tapping into Kotlin’s rich standard library, you’re missing out on a ton of useful functionality. Kotlin comes with a bunch of built-in functions that can make your code cleaner and easier to read.
- For instance, instead of writing manual null checks, you can use the let function to run a block of code only if the value is non-null. Similarly, Kotlin provides functions like apply, run, with, and also for more elegant code structuring.
By leveraging these functions, you can reduce boilerplate code and make your logic easier to follow.
Mistake 8: Ignoring Code Style Guidelines
We get it—style guides can feel like unnecessary rules. But let’s be real, code readability matters, especially when you’re working in a team. Kotlin has its own coding conventions, and ignoring them can make your code harder to maintain.
- Not following standard naming conventions or ignoring proper formatting can lead to inconsistencies. For example, using snake_case instead of camelCase for variable names will confuse others (and maybe even you down the road).
It’s best to follow Kotlin’s official style guide. Not only does it make your code more readable, but it also keeps things consistent across your team.
Mistake 9: Overlooking Testing and Debugging Techniques
Finally, we come to one of the most overlooked aspects of development—testing and debugging. Writing code is great, but if you’re not testing it, you’re setting yourself up for failure.
- Many developers make the mistake of not writing unit tests or skipping debugging practices. This leads to bugs slipping into production, and trust me, you don’t want that headache.
Make it a habit to write tests for your Kotlin code and use Kotlin’s testing libraries to ensure that everything works as expected. Debugging might seem tedious, but in the long run, it saves you from bigger issues.
Conclusion
In the end, there are plenty of mistakes to avoid as a Kotlin developer, but by being mindful of these common pitfalls, you can drastically improve your code quality. Whether it’s using null safety properly, leveraging extension functions, or avoiding Java-style coding habits, the key is to fully embrace Kotlin’s features. So, keep learning, keep testing, and write clean, efficient Kotlin code. Happy coding.
You may also like :-
- Kotlin vs Flutter: Which One is Better in 2024?
- JavaScript vs Python: Understanding the Key Differences
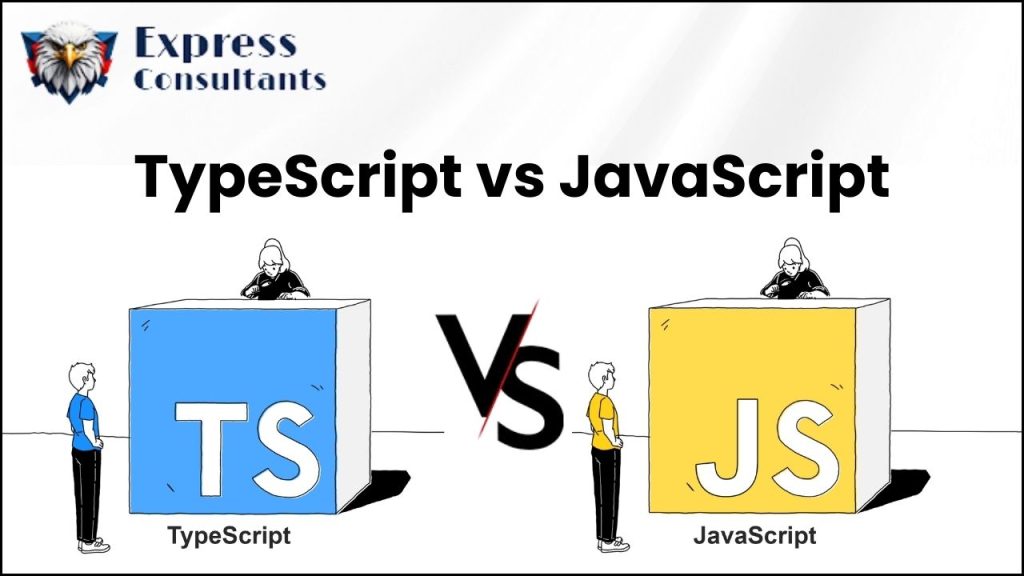
TypeScript vs JavaScript: Which One Should You Choose?
TypeScript vs JavaScript: Which One Should You Choose?
From the humble beginnings as a scripting language to becoming the backbone of web development, JavaScript has done it all. However, in recent years, TypeScript has entered the scene, promising to take JavaScript’s strengths and iron out some of its wrinkles. So, if you’re standing at the crossroads between TypeScript vs JavaScript, which path should you take?
Let’s dive in and figure out which language makes the most sense for your project.
What is JavaScript?
JavaScript, the language that powers the web, it has been around since 1995. Originally developed by Netscape, it was designed as a lightweight scripting language to add interactivity to web pages. Fast forward to today, JavaScript has evolved into one of the most popular and versatile programming languages, not just for web development but for backend (thanks to Node.js), mobile apps, and even games.
Use cases: JavaScript was initially built to run on the client side (in your browser), but with the rise of Node.js, it can also handle server-side tasks. This makes JavaScript a full-stack language, used by developers to create everything from interactive website features to robust server infrastructures.
Strengths of JavaScript:
- Flexibility: You can run JavaScript anywhere – in browsers, on servers, or even in databases like MongoDB.
- Massive community support: It’s hard to beat JavaScript in terms of library support and frameworks. React, Angular, Vue? All thanks to JavaScript.
- Fast iteration: Its dynamic nature makes prototyping fast and easy.
However, it’s not all roses. One big limitation of JavaScript is its lack of strict type checking. The language is dynamically typed, which can lead to unexpected runtime errors. This flexibility can be both a strength and a weakness, depending on your project’s complexity. You often don’t know about mistakes until your code is already running—yikes.
What is TypeScript?
Now, let’s talk about TypeScript—JavaScript’s cooler, more structured sibling. Created by Microsoft, TypeScript is a statically typed superset of JavaScript. What does that mean? Simply put, TypeScript extends JavaScript by adding optional static typing.
Key Features of TypeScript:
- Static typing: TypeScript allows you to define variable types (e.g., strings, numbers, etc.), catching potential errors early on.
- Interfaces and classes: TypeScript adds object-oriented features like interfaces and classes, making code more structured.
- Enhanced IDE support: One of the biggest advantages of TypeScript is the improved developer experience. With better autocompletion, refactoring tools, and error-checking right in your editor, it feels like a safety net for coding.
TypeScript doesn’t replace JavaScript; it just builds on top of it. Once you’ve written your code in TypeScript, the TypeScript compiler transpiles it into plain JavaScript, which browsers or servers can run. So, you’re essentially writing JavaScript with a stronger structure.
Why go through all that trouble?
Well, in larger projects or teams, TypeScript shines because it makes your codebase more predictable. You’re less likely to encounter a bug caused by a typo or incorrect variable type. While JavaScript allows you to be loose with your data, TypeScript holds you accountable, which can save a lot of debugging time down the road.
Key Differences Between TypeScript and JavaScript
When comparing TypeScript vs JavaScript, the main difference lies in how structured they are.
Key Differences Between TypeScript and JavaScript | ||
Feature | JavaScript | TypeScript |
Typing | Dynamic typing (types are inferred at runtime) | Static typing (types are defined at compile time) |
Type Safety | No type safety; types are dynamically assigned | Strong type safety; types are explicitly defined and checked during compilation |
Error Checking | Errors occur at runtime | Errors are caught earlier during code compilation (compile-time) |
Tooling and IDE Support | Basic autocompletion, limited error checking | Enhanced autocompletion, strong error checking, and better refactoring |
Learning Curve | Easier to learn; less setup | Slightly more complex due to static typing and tooling setup |
Development Speed | Faster for smaller projects and quick prototypes | Slower due to type annotations, but reduces long-term maintenance |
Use Cases | Ideal for small to medium apps, quick iterations | Best for large-scale apps, complex projects with many developers |
Transpilation | Direct execution in browsers | Needs to be transpiled (converted) to JavaScript before execution |
Community Support | Huge community, vast ecosystem of libraries and frameworks | Growing community, popular in enterprises, and strong support for modern projects |
Maintenance | Can get harder to maintain in large projects due to lack of type safety | Easier to maintain in large codebases due to predictable types and early error detection |
Code Readability | More flexible and concise, but may become harder to understand in large projects | More wordy but makes code clearer and easier to follow, especially in complex applications |
Backward Compatibility | Directly supports older browsers and environments with minimal setup | Needs to be transpiled to JavaScript, though this allows use of modern features in older browsers |
Refactoring | Refactoring can be error-prone without type information | Safer due to strong typing, making it easier to detect issues early when modifying large codebases |
Code Complexity | Flexible, but can become messy in large projects due to dynamic typing | More structured with types, resulting in cleaner, more maintainable code over time |
Type Safety: One of the biggest differences is how each handles types. JavaScript is dynamically typed, meaning types are inferred during runtime. This can lead to unexpected behavior if you don’t catch errors early. TypeScript, on the other hand, uses static typing, allowing you to define types in advance and catch potential issues before your code runs, improving overall reliability.
Tooling and IDE Support: TypeScript has a huge edge here. Because of its static types, IDEs like Visual Studio Code can provide better autocompletion, inline error detection, and easier refactoring. JavaScript, while still supported by most IDEs, doesn’t offer the same level of predictive help since types are only known at runtime.
Learning Curve: For beginners, JavaScript’s flexibility makes it easier to pick up and start coding quickly and If you know JavaScript, picking up TypeScript is a breeze, but TypeScript introduces more complexity, particularly with its static typing and configuration, that complexity pays off in long-term stability and maintainability—especially in larger projects.
Error Checking: JavaScript checks for errors while the code is running (runtime errors), which can make debugging a nightmare, especially in large applications. TypeScript, with its compile-time error detection, allows you to catch most bugs early, saving time during the development process.
Code Readability: JavaScript can become messy as projects scale due to its lack of enforced structure, whereas TypeScript’s type annotations make the code more readable and easier to maintain in the long run. The tradeoff? TypeScript tends to be more wordy because of these annotations, but many developers find the added clarity worth it.
Development Speed: If you’re working on a small or fast-moving project, JavaScript’s minimal setup and flexibility can save you time. However, TypeScript, while initially slower to set up due to the need for types and transpiling, pays off in larger applications by reducing the likelihood of bugs and making future maintenance smoother.
Refactoring and Maintenance: TypeScript shines in refactoring, as it ensures that type-related issues are caught early. If you’re working in a large team or on a project that’s expected to grow, TypeScript can help you make safer changes without breaking your code. JavaScript, without type information, can make refactoring riskier and more prone to runtime errors.
Pros and Cons of JavaScript and TypeScript
JavaScript:
Pros:
- Ubiquity: JavaScript is everywhere. Every browser runs it natively, and it has a massive ecosystem.
- Ease of learning: You can jump into JavaScript with minimal setup and quickly start building applications.
- Rich tooling: Whether it’s Node.js for the backend or frameworks like React for the frontend, JavaScript has a rich set of tools and libraries.
Cons:
- Lack of type safety: Errors due to misused types are only caught at runtime.
- Harder to maintain in large codebases: As your project grows, tracking down bugs can become a nightmare, especially in loosely typed code.
TypeScript:
Pros:
- Type safety: Bugs are caught during development rather than in production.
- Better tooling: Thanks to static types, IDEs provide better autocompletion, navigation, and refactoring capabilities.
- Scalability: For larger projects with multiple developers, TypeScript ensures that everyone adheres to a consistent coding style, reducing future headaches.
Cons:
- Learning curve: For beginners, TypeScript can feel overwhelming compared to the free-flowing nature of JavaScript.
- Compilation step: Unlike JavaScript, which runs directly in the browser, TypeScript needs to be transpiled into JavaScript before it can run in the browser.
When to Use JavaScript?
So when should you stick with JavaScript? If you’re building something small, quick, and you want to prototype fast—JavaScript might just be your best bet. It’s also ideal when you’re working on projects where strict type checking isn’t as crucial, like simple websites or small server-side applications with Node.js.
Moreover, JavaScript works great if you’re trying to use the latest frameworks and libraries without worrying about an additional compilation step. Since every browser runs JavaScript natively, you don’t have to deal with the overhead of converting TypeScript to JavaScript.
When to Use TypeScript?
On the flip side, if you’re working on a large project, with multiple contributors, and want to minimize future bugs, TypeScript is a no-brainer. Especially when the codebase becomes massive, TypeScript adds a layer of structure and predictability, making maintenance much easier. Projects like Angular, which natively supports TypeScript, or teams that deal with complex business logic often lean towards TypeScript for its robustness.
Besides, TypeScript is a great choice for developers who like catching errors during coding rather than waiting for runtime failures. With the increasing support of TypeScript across major libraries and frameworks, it’s becoming easier to integrate into various projects.
Conclusion
In the end, whether you choose TypeScript or JavaScript it depends on your project’s needs. JavaScript is fast, flexible, and ideal for smaller, simpler applications. Meanwhile, TypeScript offers safety, structure, and maintainability for larger, more complex projects.
If you’re just starting, JavaScript will get you up and running quickly. But if you’re planning to scale or build something more robust, TypeScript is the way to go. So, which one will you choose?
You may also like : –
- MERN Stack vs MEAN Stack: Which One Should You Choose?
- Why is Front-End Development Important: Why It’s Key to User Experience?
- Angular vs ReactJS: Which One is Better for Frontend Development Framework in 2024?
FAQ: TypeScript vs JavaScript
1. Do I need to learn JavaScript before TypeScript?
Yes, since TypeScript is a superset of JavaScript, it’s a good idea to have a solid grasp of JavaScript first. Think of TypeScript as an upgraded version of JavaScript—it adds extra features, but all your JavaScript knowledge will still apply.
2. Is TypeScript better than JavaScript?
Better? Well, it depends. TypeScript offers more structure and catches errors earlier, which makes it awesome for larger projects. But if you’re building something quick and simple, JavaScript’s flexibility might be more your style. It’s all about what fits your project’s needs.
3. Can TypeScript replace JavaScript?
code needs to be converted (or “transpiled”) into JavaScript before it runs. So, TypeScript is more like a helpful assistant to JavaScript.
4. Is TypeScript harder to learn than JavaScript?
TypeScript adds a layer of complexity because of static typing and other features, but if you’re already comfortable with JavaScript, you won’t find it too difficult. It just requires a bit more attention to detail, especially when defining types.
5. Should I use TypeScript for every project?
Not necessarily. TypeScript is fantastic for larger projects where you want to avoid messy bugs and improve code maintainability. But for smaller, quick projects? JavaScript’s simplicity might save you time. Use TypeScript when you want more structure and long-term stability.
6. Can I use JavaScript libraries in TypeScript?
Since TypeScript is a superset of JavaScript, you can use all the JavaScript libraries you know and love in a TypeScript project. Plus, many popular libraries already have TypeScript definitions, so you get the bonus of type safety.
7. What’s the biggest advantage of TypeScript over JavaScript?
One word: safety. TypeScript’s static typing allows you to catch potential errors early in development, making your code more predictable and easier to maintain, especially in large projects. It’s like having a spell-checker for your code.
8. Will learning TypeScript make me a better JavaScript developer?
Definitely, TypeScript forces you to think more carefully about the structure and types in your code, which can sharpen your JavaScript skills. Once you start using TypeScript, you’ll appreciate how much it improves your coding discipline.
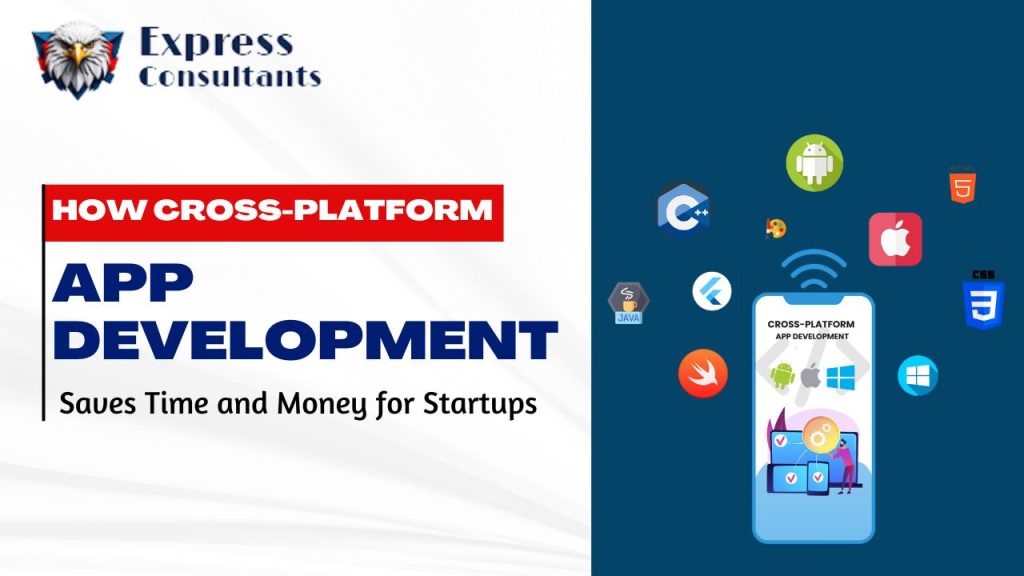
How Cross-Platform App Development Saves Time and Money for Startups
How Cross-Platform App Development Saves Time and Money for Startups
Developing for all platforms doubles your costs. Cross-platform solutions? They cut that by half.
Startups, with their relentless pursuit of innovation, often face a common dilemma: how do you launch an app that works seamlessly across devices without burning through your entire budget? The answer, increasingly, lies in cross-platform app development. Not only is it a smarter way to save time, but it’s also an absolute game-changer when it comes to saving money. Let’s break down what cross-platform app development really is and why it’s the go-to solution for startups.
What is Cross-Platform App Development?
In a nutshell, cross-platform app development is like making one app that fits all. Instead of building separate versions for iOS, Android, and other operating systems, you code once and deploy it across multiple platforms. How sweet does that sound? Imagine the amount of time you save when you don’t need to build and maintain multiple versions of the same app.
So yeah, with a single codebase, your app gets a wider reach without the overhead of developing and maintaining different versions. Developers use tools like Flutter, React Native, or Xamarin to create these apps, allowing startups to get the most for their buck without worrying about users on different devices being left out.
Benefits of Cross-Platform App Development for Startups
Now, let’s talk benefits—’cause we all love those, right? Cross-platform app development has a bunch, especially for startups looking to stretch every dollar and make every second count.
- Cost Efficiency: Instead of paying developers to write code for multiple platforms, you’re paying for one team to develop a single app that works everywhere. That’s a no-brainer, According to research, businesses save around 30-40% on costs when they opt for cross-platform development over native development.
- Faster Time to Market: Time is money, particularly for startups trying to get ahead of the competition. With a single codebase, the time taken to launch on multiple platforms is drastically reduced. You can get your app out there, start gaining traction, and pivot if necessary—faster.
- Easier Maintenance & Updates: When you need to push updates or fixes, you do it once. Just once, this keeps things simple and ensures consistency across all platforms. Imagine managing two separate development tracks—sounds like a nightmare, right?
- Wider Reach with Consistency: Whether your user has an iPhone or a Samsung, your app’s experience stays consistent. This uniformity can build brand loyalty faster because people love when things work seamlessly, no matter what device they’re using.
Specific examples? Take Instagram and Uber Eats. Both started using cross-platform solutions to scale their services more quickly across multiple devices, and look where they are now.
Choosing the Right Cross-Platform Development Framework
So, you’ve decided to go cross-platform. But which framework should you choose? That’s where it gets a bit tricky. Picking the right framework isn’t just about what’s popular, it’s about what suits your specific needs.
- Flutter: Created by Google, this one’s quickly gaining steam, especially if you want a slick, native look and feel without the hassle of native code.
- React Native: Facebook’s baby and one of the most popular frameworks out there. Tons of startups love React Native for its efficiency and ease of use.
- Xamarin: Part of the Microsoft family, Xamarin is ideal if you’re already knee-deep in .NET.
But hey, don’t let this list overwhelm you, the key is to align the framework with your project’s goals, the skillset of your developers, and the experience you want to deliver. Trust me, you don’t wanna spend weeks coding in a framework only to realize halfway that it doesn’t suit your app’s needs. That’s just bad juju.
Potential Drawbacks and How to Overcome Them
Alright, no sugar-coating here—cross-platform development isn’t all sunshine and rainbows. It’s got its drawbacks, but, as with anything, knowing how to handle them makes all the difference.
- Performance Issues: Since cross-platform apps are designed to run on multiple devices, they may not be as fast or optimized as native apps. The key is to work with an experienced developer who knows how to optimize performance using best practices.
- Limited Access to Native Features: Sometimes, cross-platform frameworks don’t give you full access to device-specific features (like the latest iOS updates). But hey, there’s a workaround. You can implement native code alongside cross-platform solutions, a little hybrid magic, if you will.
- UI/UX Consistency: It’s trickier to achieve the exact native look for each platform, but again, a good designer knows how to handle these nuances.
How Express Consultants Can Help You in Cross-Platform App Development
Here’s where Express Consultants comes in, and trust me, we know our stuff. We’re all about helping startups get the most out of cross-platform app development by taking a highly tailored approach.
Unlike a lot of agencies that slap together an app and call it a day, Express Consultants dives deep into understanding what your startup truly needs. We’ll help you choose the right framework, ensure your app performs like a beast across all devices, and most importantly, we make sure you stay on budget.
But that’s not all. Our team doesn’t just hand you an app and walk away. Nope. We’re with you for the long haul, making sure the app stays updated, optimized, and competitive in the ever-evolving mobile market.
Wrapping It All Up
To sum it up, cross-platform app development offers startups a fast, cost-effective way to reach a broad audience without the headache of managing multiple codebases. Whether it’s saving time, reducing costs, or ensuring consistent performance across devices, it’s a solution that startups just can’t ignore.
And when it comes to making this happen, companies like Express Consultants have your back. So, if you’re ready to save some serious cash, get your app to market faster, and still deliver a quality product, why not give cross-platform app development a shot?
In the end, choosing this path isn’t just about saving money—it’s about making smart, scalable decisions that keep your startup ahead of the competition. Ready to build the next big thing? Let’s make it happen with cross-platform development.
FAQ
1. What exactly is cross-platform app development?
In simple terms, cross-platform app development lets you build one app that works across multiple platforms (like iOS and Android) using a single codebase. Instead of creating separate apps for each platform, developers use frameworks like Flutter or React Native to make sure the app runs smoothly on all devices.
2. Why should startups consider cross-platform development?
Startups are always looking to save time and money, right? Cross-platform development lets them do exactly that. Instead of hiring separate teams to build different versions of the app for each platform, you can get the job done with one team.
3. How does cross-platform app development save money?
Since you only need to build one codebase that works across all platforms, you’re cutting down on development costs big time. Maintenance is easier too—updates only need to be done once. No need to worry about juggling multiple versions of the same app. That’s a huge money-saver.
4. Will a cross-platform app perform as well as a native app?
For most cases, yes, While there might be slight performance differences, they’re usually small enough that users won’t even notice. With the right developers and optimization, cross-platform apps can perform really well—just like their native counterparts.
5. What are some potential drawbacks of cross-platform development?
A couple of things: cross-platform apps may not access every native feature of a device, and performance might not be as fast as native apps in very specific cases. But honestly, these issues can often be worked around by skilled developers or using hybrid methods (adding a bit of native code where necessary).
6. Which cross-platform framework is best for startups?
It really depends on your specific needs. For instance:
- Flutter (from Google) is great for creating apps that look native and feel smooth.
- React Native (Facebook’s tool) is a popular choice for startups because it’s user-friendly and gets the job done fast.
- Xamarin (from Microsoft) is best if you’re already using .NET.
Each one has its perks—choosing the right one comes down to what kind of app you’re building and your team’s expertise.
7. Can cross-platform development help me launch my app faster?
Absolutely, with just one codebase to manage, development time is significantly reduced. You’ll get your app on both Android and iOS simultaneously, allowing you to enter the market quicker than if you were developing separate apps for each platform.
8. What if my app needs to use specific features from iOS or Android?
No worries, even though cross-platform apps don’t always have full access to platform-specific features, you can still add native code where needed. This “hybrid” approach gives you the best of both worlds—wide compatibility and specific functionality when required.
9. How does cross-platform development impact updates and maintenance?
One of the best things about cross-platform development is that updates only need to be done once. This makes maintaining and upgrading your app so much easier. You won’t have to worry about separate teams working on different versions—it’s all streamlined into one process.
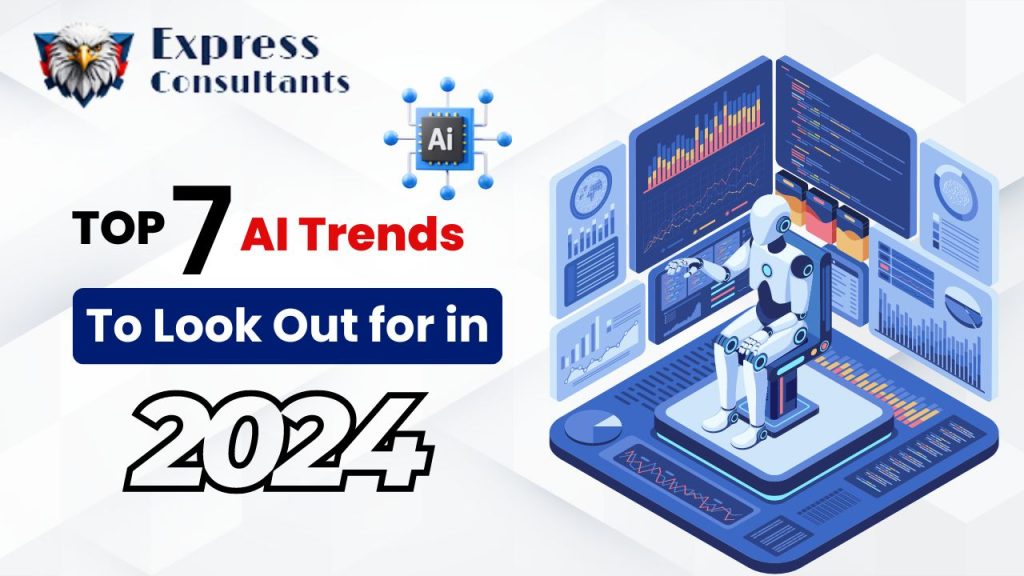
Top 7 AI Trends To Look Out for in 2024
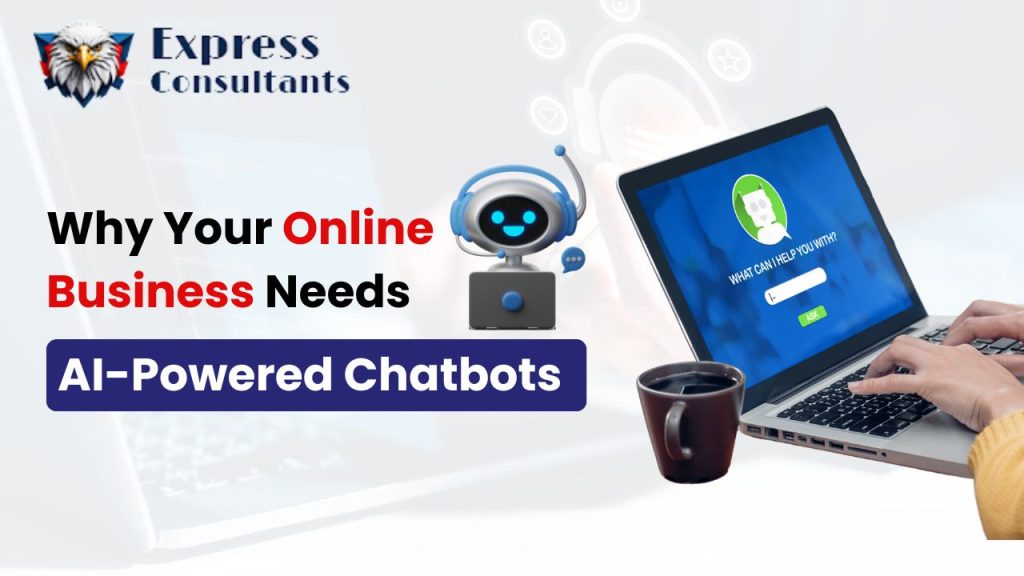
Why Your Online Business Needs AI-Powered Chatbots
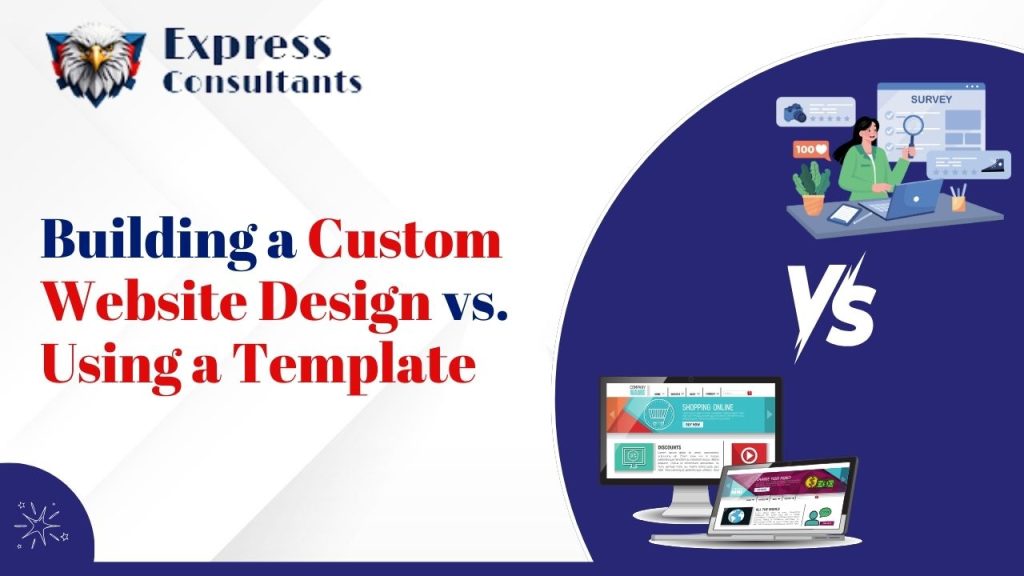
Building a Custom Website Design vs. Using a Template: Which One Is Right for You?
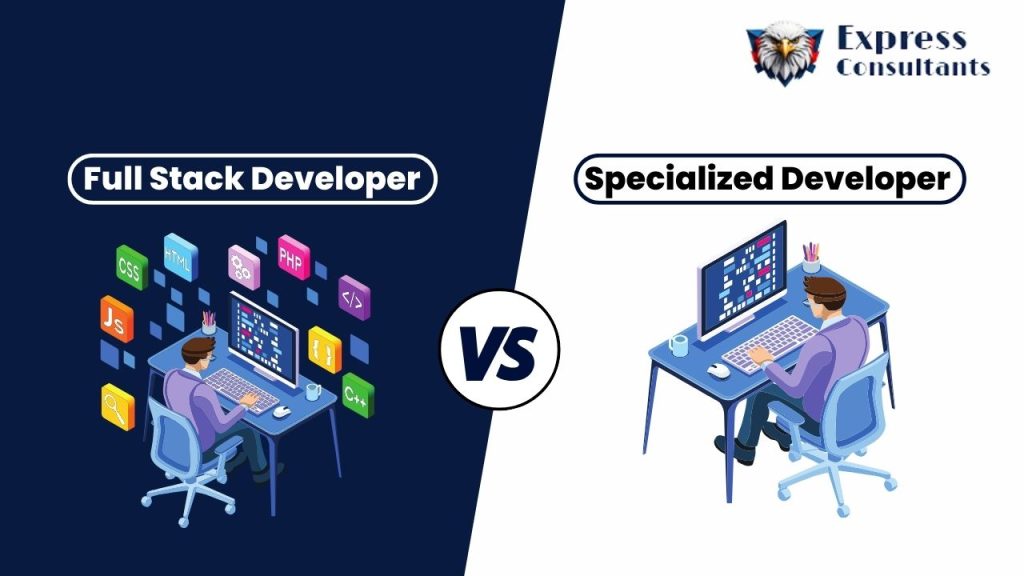
Full Stack Developer vs. Specialized Developer: Which is Right for Your Project?
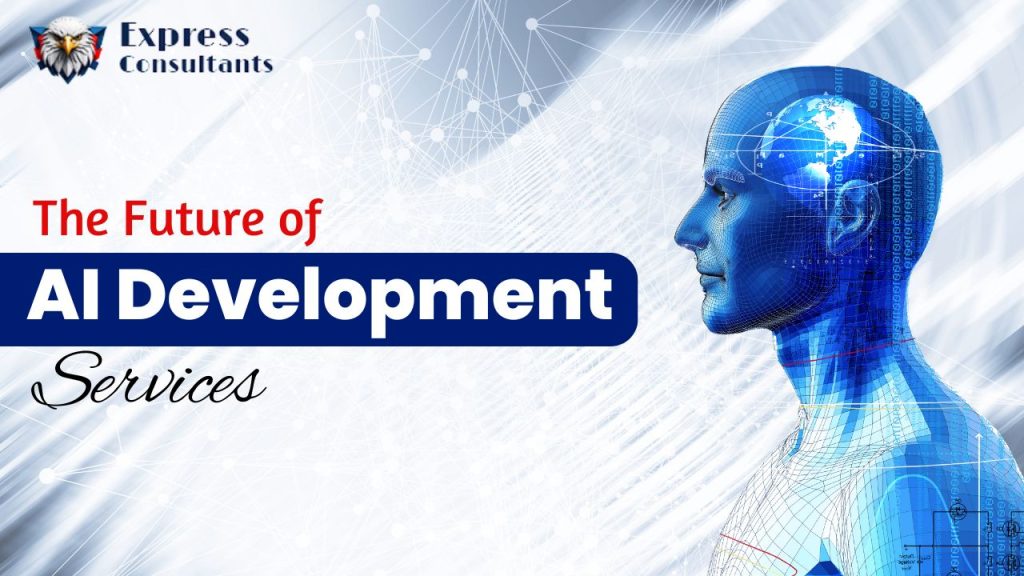
The Future of AI Development Services: Predictions for 2025 and Beyond
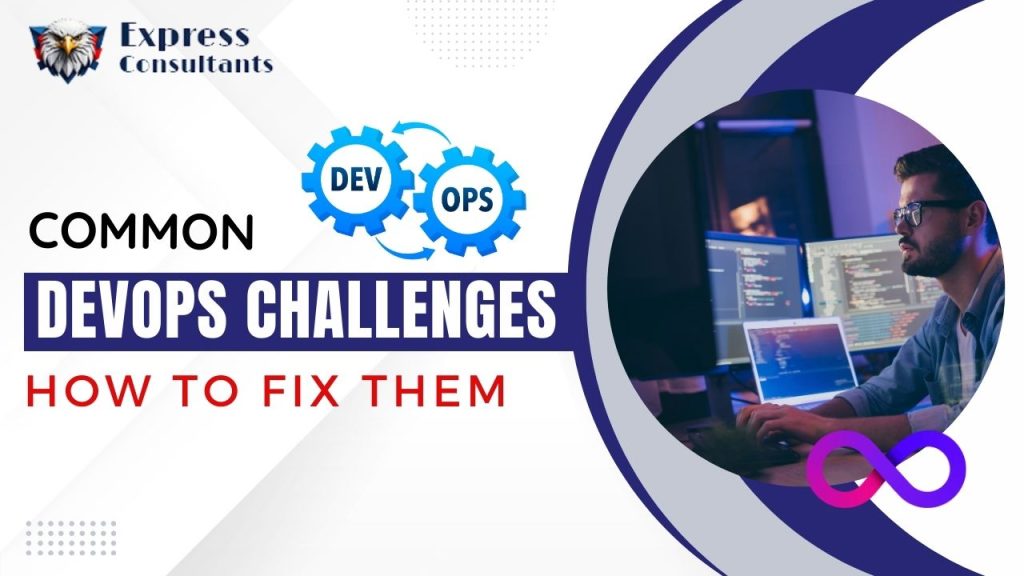
Common DevOps Challenges and How to Fix Them
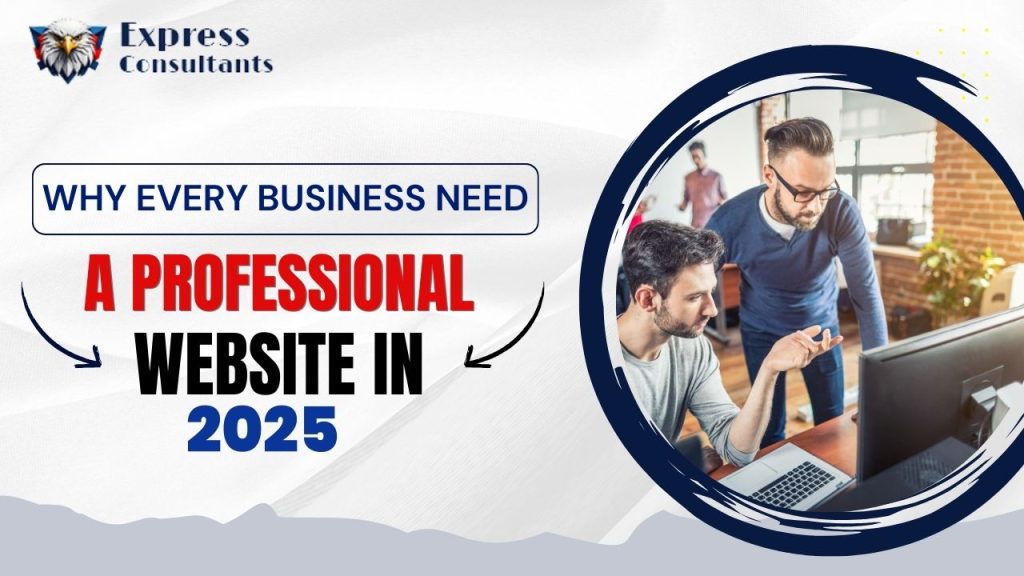