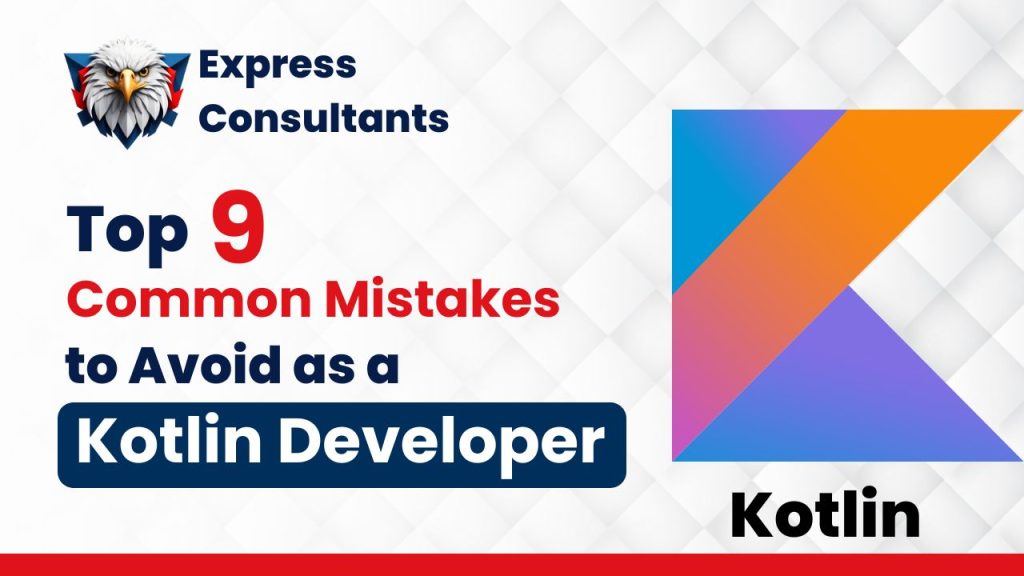
Top 9 Common Mistakes to Avoid as a Kotlin Developer
October 20, 2024Top 9 Common Mistakes to Avoid as a Kotlin Developer
“By failing to prepare, you are preparing to fail.” – Benjamin Franklin. When it comes to coding in Kotlin, a lack of awareness about the language’s features can be just as damaging as skipping preparation altogether. Kotlin is one of the most popular languages for Android development, but that doesn’t mean every developer uses it to its full potential. Whether you’re transitioning from Java or just diving into Kotlin, it’s easy to make mistakes that hinder your progress. Let’s dive into some common mistakes to avoid as a Kotlin developer, so you can write better, cleaner code.
Mistake 1: Ignoring Null Safety Features
Kotlin’s null safety is one of its most touted features. It basically takes away the headache that most of us have from dealing with NullPointerException in Java. But believe it or not, some devs still manage to overlook this powerful feature. Sounds wild, right?
- In Kotlin, you have to specifically mark a variable as nullable by adding a ? to its type. If you’re not using this feature, you’re asking for trouble. Why? Because you’re treating Kotlin like Java, ignoring one of the very things that makes it better.
- Skipping null safety can result in bugs that are hard to debug and can cause unexpected crashes. For example, instead of handling nullables with care, developers might assume everything will work fine, only to get bitten by the dreaded runtime errors.
So, next time you write code, make sure you fully embrace Kotlin’s null safety tools like safe calls (?.), the Elvis operator (?:), and non-null assertions (!!).
Mistake 2: Overusing the !! Operator
The !! operator can be a double-edged sword. It’s tempting, I know. You see that pesky null, and you just slap a !! in there to make it disappear. Boom! Problem solved, right? Wrong. Overusing this operator is like playing with fire.
- The !! operator tells Kotlin, “I know this value isn’t null, let me handle it!” However, if the value is indeed null, guess what? Your app will crash with a KotlinNullPointerException. Essentially, it’s like running with scissors. Sure, you can do it, but you’re risking unnecessary issues.
Instead of recklessly throwing in !!, use safe calls and proper null checks. Kotlin gives you tools to manage nullability properly, so use them wisely.
Mistake 3: Not Using Extension Functions
Ah, extension functions. These bad boys are one of Kotlin’s coolest features, yet many developers forget to take full advantage of them. Extension functions let you extend the functionality of classes without modifying their source code. That’s pretty neat, right?
- For example, if you frequently need to transform a string to title case, instead of rewriting the same code everywhere, you can create an extension function:
fun String.toTitleCase(): String {
return this.split(” “).joinToString(” “) { it.capitalize() }
}
See? Now you can call .toTitleCase() on any string, making your code cleaner and more readable. Extension functions are an easy way to DRY (don’t repeat yourself) up your code. Don’t skip ‘em!
Mistake 4: Neglecting Data Classes
Data classes are your go-to when you just need to hold data. Kotlin makes this super easy with the data keyword, but somehow, devs still forget to use it!
- If you’re writing a class that’s just meant to hold data and you’re manually writing equals(), hashCode(), or toString() methods, you’re doing it wrong. Data classes automatically provide these methods, saving you tons of time and effort.
Why reinvent the wheel? Just declare a class as a data class, and Kotlin does the heavy lifting for you.
Mistake 5: Misusing Coroutines
Coroutines are what makes Kotlin a real treat for asynchronous programming, but they can easily be misused if you’re not careful. One common mistake? Launching coroutines on the wrong dispatcher or not managing them properly.
- Developers might launch coroutines on the main thread, resulting in UI blocking or slow performance. To avoid this, make sure you launch coroutines on the correct dispatcher, such as Dispatchers.IO for network or disk operations.
Coroutines are a fantastic tool, but if you misuse them, you might end up with unresponsive UIs or unexpected crashes. Always be mindful of where and how you’re launching your coroutines.
Mistake 6: Writing Java-Style Kotlin Code
Kotlin and Java are close relatives, but writing Kotlin like it’s Java? Big mistake. Many developers transitioning from Java tend to write Java-esque code in Kotlin. This defeats the purpose of using a modern, concise language like Kotlin.
- For example, using traditional loops (for loops) instead of taking advantage of Kotlin’s collection functions like map, filter, or forEach. Why write verbose code when Kotlin allows you to express it more succinctly?
Kotlin is designed to be expressive and concise, so don’t fall into the trap of writing Java-style code.
Mistake 7: Not Leveraging Kotlin’s Standard Library
If you’re not tapping into Kotlin’s rich standard library, you’re missing out on a ton of useful functionality. Kotlin comes with a bunch of built-in functions that can make your code cleaner and easier to read.
- For instance, instead of writing manual null checks, you can use the let function to run a block of code only if the value is non-null. Similarly, Kotlin provides functions like apply, run, with, and also for more elegant code structuring.
By leveraging these functions, you can reduce boilerplate code and make your logic easier to follow.
Mistake 8: Ignoring Code Style Guidelines
We get it—style guides can feel like unnecessary rules. But let’s be real, code readability matters, especially when you’re working in a team. Kotlin has its own coding conventions, and ignoring them can make your code harder to maintain.
- Not following standard naming conventions or ignoring proper formatting can lead to inconsistencies. For example, using snake_case instead of camelCase for variable names will confuse others (and maybe even you down the road).
It’s best to follow Kotlin’s official style guide. Not only does it make your code more readable, but it also keeps things consistent across your team.
Mistake 9: Overlooking Testing and Debugging Techniques
Finally, we come to one of the most overlooked aspects of development—testing and debugging. Writing code is great, but if you’re not testing it, you’re setting yourself up for failure.
- Many developers make the mistake of not writing unit tests or skipping debugging practices. This leads to bugs slipping into production, and trust me, you don’t want that headache.
Make it a habit to write tests for your Kotlin code and use Kotlin’s testing libraries to ensure that everything works as expected. Debugging might seem tedious, but in the long run, it saves you from bigger issues.
Conclusion
In the end, there are plenty of mistakes to avoid as a Kotlin developer, but by being mindful of these common pitfalls, you can drastically improve your code quality. Whether it’s using null safety properly, leveraging extension functions, or avoiding Java-style coding habits, the key is to fully embrace Kotlin’s features. So, keep learning, keep testing, and write clean, efficient Kotlin code. Happy coding.
You may also like :-
- Kotlin vs Flutter: Which One is Better in 2024?
- JavaScript vs Python: Understanding the Key Differences
Ready to unlock your business's potential with custom software
Contact Us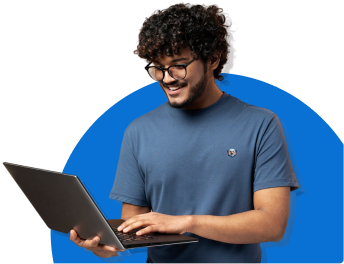